Managing socket connections and room transitions in React.js
To use socket connections in different pages of an application for different purposes, you can set up a single socket connection in a central location and reuse it across different components or pages of your application. This is particularly useful in real-time applications using libraries.
When building applications like chat systems or real-time reaction counters in React using Socket.io, managing socket connections and room transitions becomes critical, especially when different components need to interact with the same or different rooms. Here’s how to effectively handle socket connections across multiple pages, ensuring smooth transitions between them.
Challenges
- Multiple Socket Instances: If you use socket connections in different pages in an application for different purposes like using socket connections for a social media page with multiple entries for posting reactions and in a chat page for showing instant comments, initialising multiple socket connections can lead to issues like disconnections or conflicting socket events.
- Room Management: Handling room transitions (e.g., joining a room for media-based interactions and leaving it when moving to chat-based rooms) can cause issues if not managed properly. You might see stale room listeners or unintended room joins with wrong IDs.
- Rejoining Rooms with Incorrect IDs: A common issue is joining rooms with an incorrect identifier (like using
mediaId
in a chat room instead ofroomId
).
Solutions
- Single Socket Instance: Ensure that you use one persistent socket instance throughout the application, rather than creating new socket instances on every page load. This can be managed by using a central
socketService.js
. Example for socketservice.js is shown below.
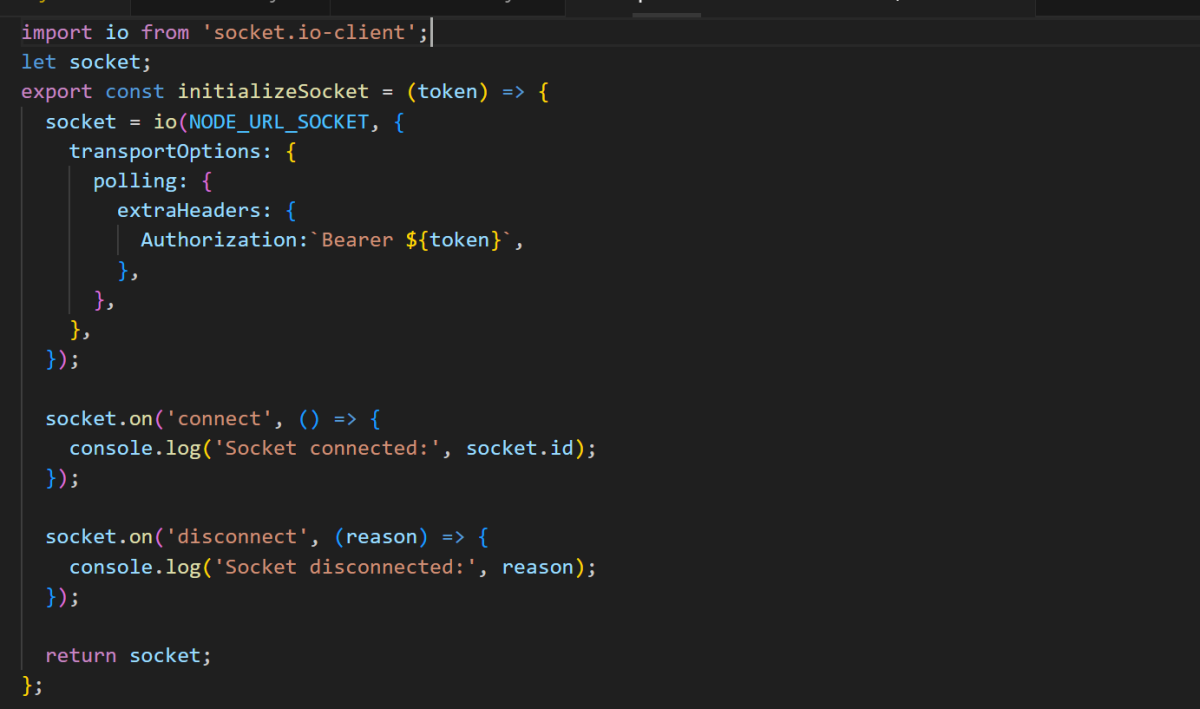
- Separate Room Logic:
For components likeEmojiCounter
(reactions), join and leave rooms usinga suitable identifier
.
Example for EmojiCounter shown below.
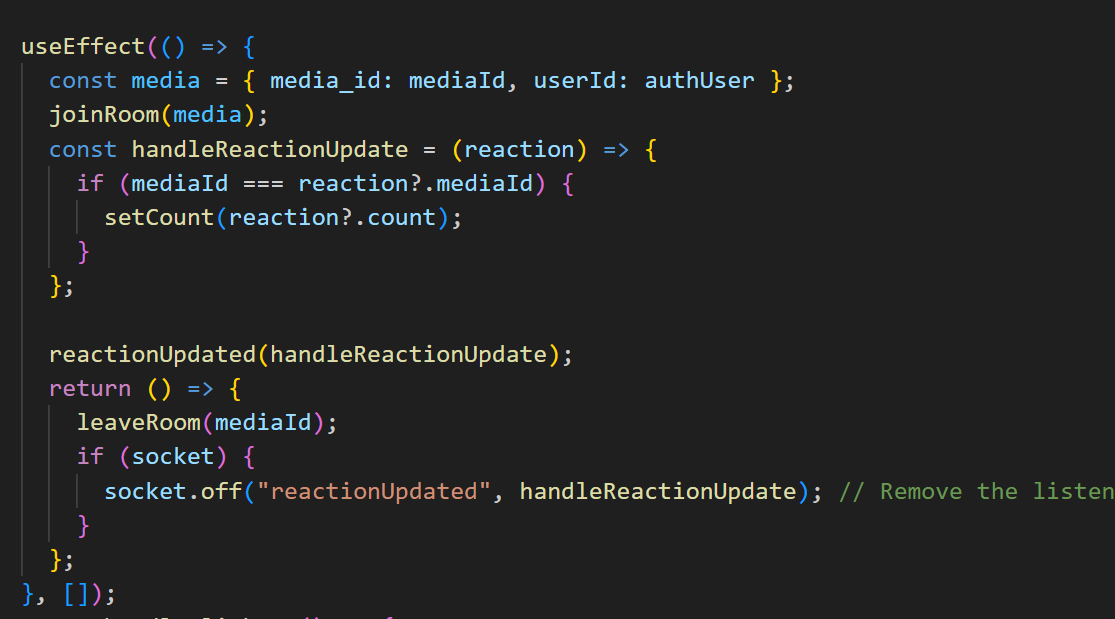
For chat-related components, use a different identifier. Below is the example.
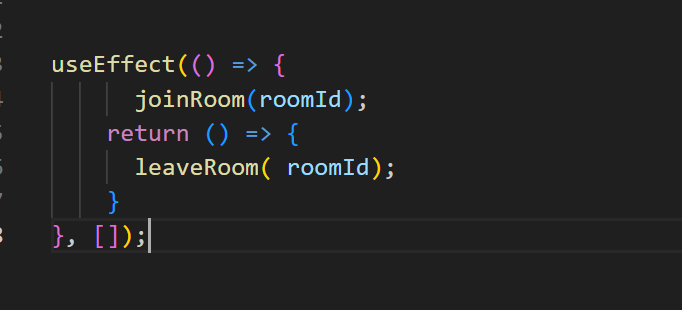
- Proper Listener Cleanup: Always remove event listeners when components unmount to avoid memory leaks or multiple handlers being triggered unintentionally. Use
socket.off()
in the cleanup function withinuseEffect
.
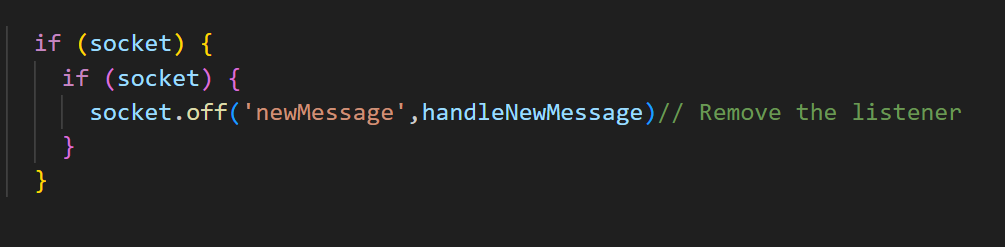
Key Takeaways:
- Maintain a single socket connection across the application to prevent disconnection issues.
- Separate room joining logic based on the context (
mediaId
for feed reactions,roomId
for chat). - Ensure proper room management when navigating between pages, including joining and leaving rooms cleanly.
By following these steps, you can ensure that your React app handles socket connections and room management efficiently, providing a smoother user experience across different real-time features