In this comprehensive guide, we will delve deep into mastering error handling with async/await
in JavaScript, equipping you with the skills to create robust and reliable applications.
Understanding Asynchronous Programming
Asynchronous programming enables web applications to perform multiple tasks simultaneously, enhancing performance and responsiveness. Traditional callback-based approaches often lead to callback hell and convoluted code. async/await
was introduced to address these issues and streamline asynchronous code execution.
The Essence of async/await
async/await
is a pair of keywords in JavaScript that simplifies the process of working with promises, making asynchronous code look and behave more like synchronous code. The async
keyword is used to define an asynchronous function, which returns a promise. Within a async
function, the await
the keyword is used to pause the execution of the function until a promise is resolved.
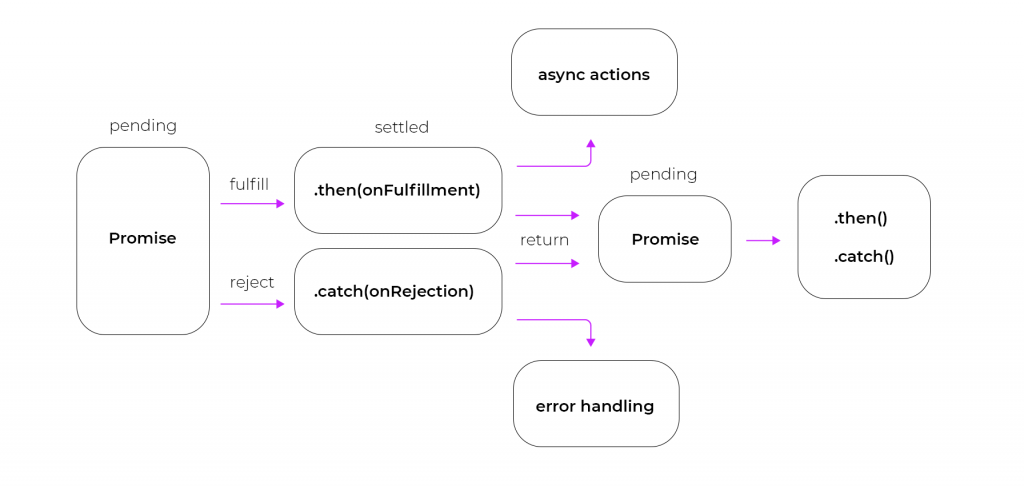
Here’s a basic example:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
return data;
} catch (error) {
console.error('Error fetching data:', error);
throw error;
}
}
Effective Error Handling with async/await
Error handling is crucial in any application to provide graceful fallbacks and user-friendly feedback. With async/await
, managing errors becomes more intuitive and concise.
Try-Catch Blocks: By encapsulating await
statements within a try
block, you can catch and handle errors that occur during asynchronous operations.
Promises Rejection: When an async
function returns a rejected promise, it automatically throws an exception, allowing you to catch and handle it in an outer try-catch
block.
Handling Multiple Promises: You can use Promise.all()
to handle multiple asynchronous operations concurrently and catch errors collectively.
Best Practices for Error Handling
- Specific Error Messages: Provide clear and concise error messages that help developers identify and troubleshoot issues quickly.
- Logging and Monitoring: Implement robust logging and monitoring mechanisms to track errors and gather insights for continuous improvement.
- Graceful Degradation: Design your application to gracefully handle errors by providing fallbacks and alternative actions for users.
Practical Implementation: Building a Weather Application
Let’s put our knowledge into practice by creating a simple weather application that fetches weather data from an API and displays it to the user. Below is a high-level overview of the steps involved:
- Setting Up the Project: Initialize a new project using a package manager like npm or yarn.
- Fetching Weather Data: Use the
fetch
API to retrieve weather data from a reliable source. - Parsing and Displaying Data: Process the fetched data and display it to the user interface.
- Error Handling: Implement robust error handling using
async/await
and provide meaningful feedback to users in case of errors.
Here’s a simplified code snippet demonstrating the error-handling approach:
async function fetchWeather() {
try {
const response = await fetch('https://api.weather.com/data');
const data = await response.json();
// Process and display data
} catch (error) {
console.error('Error fetching weather data:', error);
// Display user-friendly error message
}
}
Conclusion
Mastering error handling with async/await
in JavaScript empowers developers to build resilient and efficient applications that provide exceptional user experiences. By effectively managing asynchronous operations and gracefully handling errors, you can create codebases that are both robust and maintainable. Embrace the power of async/await
to elevate your web development skills and stay ahead in the ever-evolving landscape of modern programming.
function thisThrows() {
throw new Error("Thrown from thisThrows()");
}
try {
thisThrows();
}
catch (e) {
console.error(e);
}
finally {
console.log('We do cleanup here');
}
Summary
- We can use
try...catch
for synchronous code. - We can use
try...catch
(in combination withasync
functions) and the.catch()
approaches to handle errors for asynchronous code. - When returning a promise within a
try
block, make sure toawait
it if you want thetry...catch
block to catch the error. - Be aware when wrapping errors and rethrowing, that you lose the stack trace with the origin of the error.
Start your journey towards becoming an error-handling maestro with async/await
and watch your applications flourish.
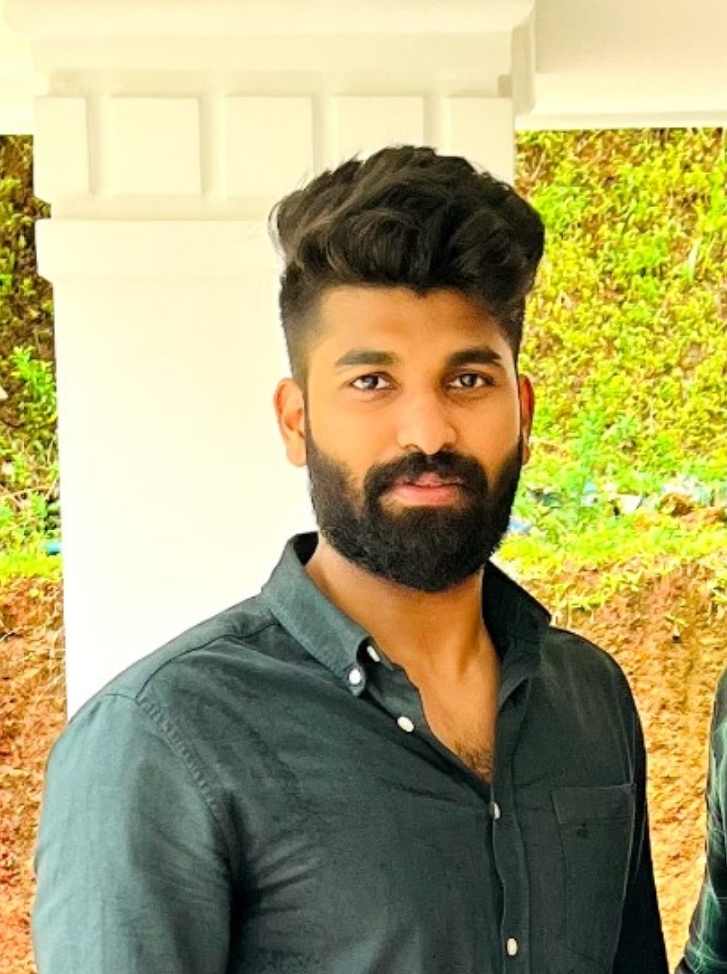