In the world of APIs, REST (Representational State Transfer) has been the dominant approach for many years. However, as applications have evolved, so have the needs of developers. Enter GraphQL a revolutionary query language for APIs that gives developers more power, flexibility, and control.
What is GraphQL?
GraphQL is a query language for APIs, it allows clients to request exactly the data they need and nothing more. In a REST API, you’re often working with predefined endpoints that return fixed data structures. If you want multiple types of data, you might need to make several requests to different endpoints. GraphQL solves this by using a single endpoint where you can ask for exactly the data you want, down to individual fields.
For example, instead of hitting different endpoints to get user data and their posts, you could do it all in one go with GraphQL:
query {
user(id: "123") {
name
posts {
title
content
}
}
}
Why Use GraphQL?
GraphQL is designed to solve some of the limitations of REST
- Efficient Data Fetching: You only request the data you need, reducing over-fetching – getting unnecessary data and under-fetching – not getting enough data.
- Multiple Resources in One Request: GraphQL allows you to fetch data from multiple related resources in one call.
- Strongly Typed Schema: You can know exactly what data is available and how to structure your queries ahead of time.
- Single Endpoint: REST APIs, require separate endpoints for different resources, GraphQL has just one endpoint for all requests. You just specify what data you want in your query.
- Better for Frontend Developers: GraphQL allows frontend developers to shape the data they need on the client-side, giving them much more flexibility and control.
GraphQL vs REST:
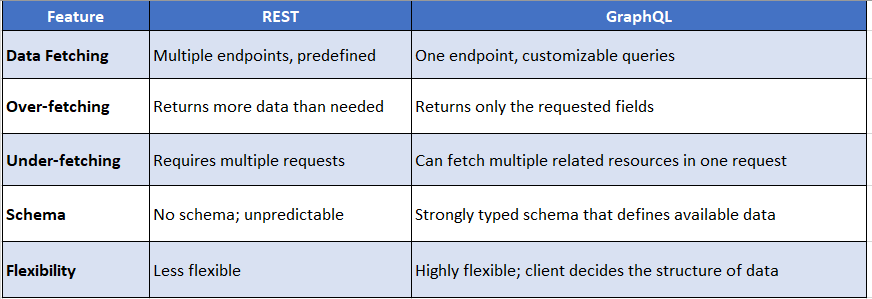
How Does GraphQL Work?
- Schema: Schema defines the structure of the data . It is like a contract between client and server, so both know what to expect.
- Queries: Queries are the requests for data. When you send a query , specify the exact fields you need, and it will give the exact data.
- Mutations: Mutation in GraphQL is a way to modify or change data on the server, such as creating, updating, or deleting records.
- Resolvers: Resolvers are functions to fetch requested data for query or mutation.
Example of a query to fetch a user’s name and email:
query {
user(id: "123") {
name
email
}
}
And here’s a mutation to add a new user:
mutation {
addUser(name: "Akash", email: "akash@example.com") {
id
name
email
}
}
Response:
{
"data": {
"addUser": {
"id": "1",
"name": "Akash",
"email": "akash@example.com"
}
}
}
Setting Up GraphQL
Setting up a GraphQL server is easy. We’ll use Node.js and Apollo Server to get started.
First, install the necessary packages:
npm install apollo-server graphql
Next, create a simple server with a good morning query:
const { ApolloServer, gql } = require('apollo-server');
// Define the schema
const typeDefs = gql`
type Query {
hello: String
}
`;
// Define the resolvers
const resolvers = {
Query: {
hello: () => 'Good Morning!',
},
};
// Start the server
const server = new ApolloServer({ typeDefs, resolvers });
server.listen().then(({ url }) => {
console.log(`🚀 Server ready at ${url}`);
});
Response:
{
"data": {
"hello": "Good Morning!"
}
}
We have done a basic GraphQL server running. Now, you can head over to GraphQL Playground to test your queries.
Note: If you are familiar with Express.js, you can use the express-graphql package, which is a simple middleware that integrates GraphQL into an existing Express app.https://graphql.org/graphql-js/running-an-express-graphql-server/
Using GraphQL Playground
GraphQL Playground is an interactive environment , where we can test our queries and mutations. It is like Postman but for GraphQL. Once your server is up, you can open the Playground in your browser and start running queries. You can use this tool to explore and test different queries and mutations as you build out your API.
Mutations in Action
Mutations are used to modify data.
Example: Suppose you want to add a new user to your database. You can do that with a mutation like this:
mutation {
addUser(name: "Aadi", email: "aadi@example.com") {
id
name
}
}
In the response, you’ll get the user’s ID and name, confirming that the user was successfully added.
Conclusion
GraphQL is a great tool that’s becoming very popular for building APIs. It gives developers more control, flexibility, and makes it easier to get the right data. Whether you’re building a small app or a large one, GraphQL helps you to fetch data and reduces the complexity of your API requests.
If you have only used REST APIs before, give GraphQL a try , You will quickly see why so many people are switching to it. Once you get used to it, you will wonder how you ever worked without it.