In the JavaScript ecosystem, Jest and Axios are powerful API testing tools that can be combined to use efficiently. Jest is a popular testing framework, while Axios is a well-known HTTP client for making requests to APIs. This article will explore how to use Jest and Axios together for API testing.
Why API Testing is Important?
Testing API ensures that the endpoints are reliable, return the correct data, and handle errors properly.
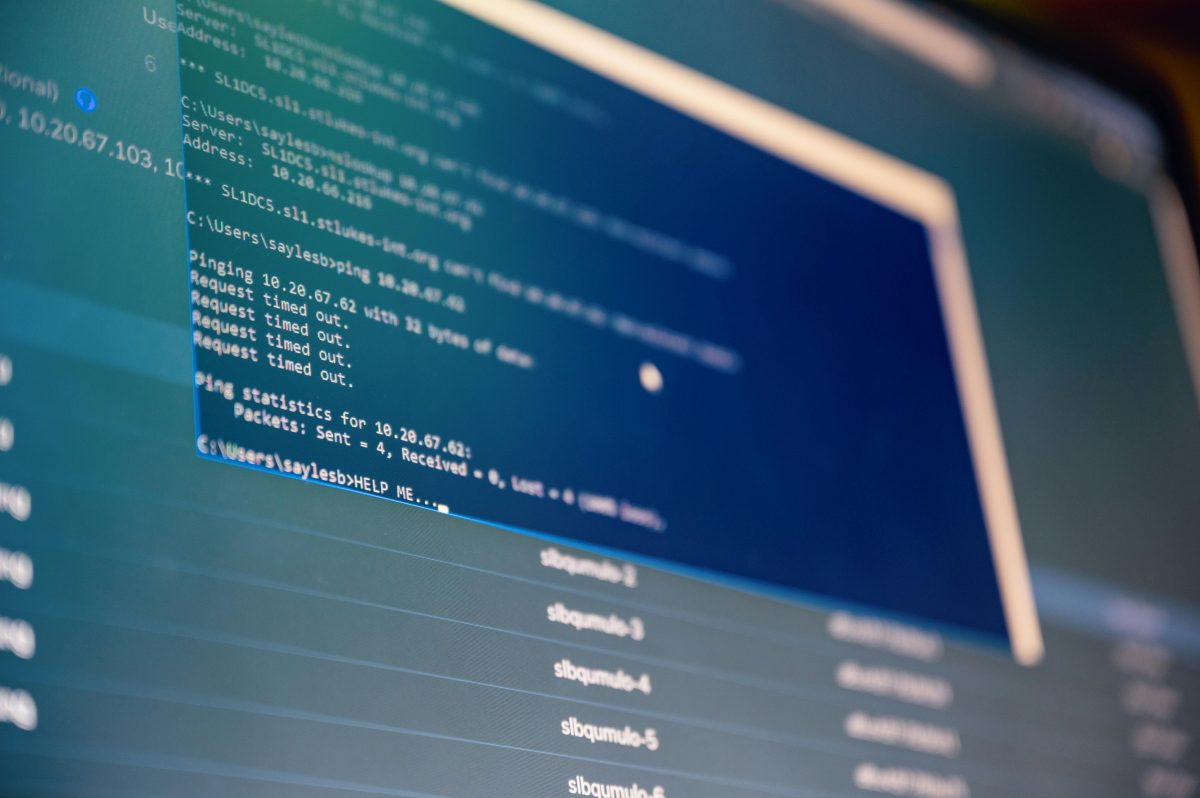
API testing using Jest vs Postman
Postman for API Testing
Postman is a specialized tool for API testing and development, widely used for both manual and automated API testing due to its robust, easy-to-use interface. However, while Postman is excellent for manual and exploratory testing, it is often used as a standalone tool, separate from the codebase. This can create an additional layer of testing that may not easily integrate with CI/CD pipelines or your main application’s test suite.
Jest for API Testing
Jest is primarily designed for unit and integration testing of JavaScript code. However, it can also be used for API testing, particularly by making HTTP requests in your tests (often combined with libraries like axios, supertest, or node-fetch).
When to Choose Postman vs Jest
- Use Postman if you are primarily performing manual API testing or need a visual interface for exploring API behavior and responses. It’s also a good choice when you want to share test collections with non-developers or collaborate on testing without needing deep code-level integration.
- Use Jest if you are focused on automated API testing as part of your JavaScript codebase, and you want to integrate API tests directly into your CI/CD pipeline. Jest is more suited for developers who want a programmatic approach to testing with the flexibility to customize their testing log. In this blog, we are focussing on using Jest for API testing.
What is Jest?
Jest is a JavaScript testing framework developed by Facebook. It is widely used for testing React applications. In order to use Jest for API testing, you’ll need to configure and manage HTTP request libraries like axios
or supertest
. Here, we are using Axios.
What is Axios?
Axios is a promise-based HTTP client that works in both the browser and Node.js environments. It simplifies making HTTP requests like GET, POST, PUT, and DELETE. Axios handles asynchronous requests using promises, making it easy to handle API responses.
Setting Up Jest and Axios
Before you begin testing, you need to install Jest and Axios in your project. Here’s how:
1. Install Jest and Axios:
Run the following command to install both packages:
> npm install --save-dev jest axios
2.Configuration object for jest
In your package.json
, add a script to run Jest tests:
"scripts": {
"test": "jest"
}
> Configuring jest.config.js
This allows you to customize Jest’s behaviour according to your project’s needs.
@type {import('jest').Config} */
const config = {
// Custom reporters for output formatting
reporters: ['default'],
// Specify a test timeout of 15 seconds
testTimeout: 15000,
// Glob patterns to find test files
testMatch: ['**/__tests__/**/*.test.js', '**/?(*.)+(spec|test).[jt]s?(x)'],
};
module.exports = config;
3. Run test script
Run the command npm run test
in the terminal
npm run test
4.Test report on HTML format
> Install the Jest HTML Reporter
To install the jest-html-reporter
package:
npm install --save-dev jest-html-reporter
> Configure Jest to Use the Reporter
configuration to include jest-html-reporter
module.exports = {
reporters: [
'default',
['jest-html-reporter', {
pageTitle: 'XXXX API-Testing Report',
dateFormat: 'dd-mm-yyyy HH:MM:ss',
includeFailureMsg: true,
outputPath: 'reports/test-report.html'
}]
],
};
After the tests are completed, an HTML report will be generated and saved in the specified outputPath
(in this case, reports/test-report.html
).
Basic API Testing with Jest and Axios
To illustrate how to use Jest and Axios together, let’s test a simple API that fetches user data. Here’s how you can write a test using GET & POST requests.
const axios = require('axios')
let user1_authTocken = null;
const api = axios.create({
baseURL:process.env.API_server,
timeout: 3000
});
test('login as client user-xyz',async () => {
const resp = await api.post('/api/v1/auth/login', {
"username": "xyz@mailinator.com",
"password": "XXXXXXXX"
});
if (resp.data.success)
user1_authTocken = resp.data.access_token;
});
test('get-projectlist',async()=> {
const resp = await api.get('/api/v1/project/get-all-project-by-email', {
headers:{
'Authorization': 'bearer '+ user1_authTocken
}
})
expect(resp.status).toBe(201);
expect(resp.data.success).toBeTruthy();
});
test.skip('list-existing-org-xxx', async () => {
const resp = await api.get('/api/app-xxxx-organisations');
console.log(JSON.stringify(resp.data, null, 4));
expect(resp.data.data.length).toBeGreaterThan(0);
});
In this test:
- Axios makes a POST request to an API that returns login token
- Next test function make use of login token to send a GET request to check the response contents and verify that the response is coming properly.
- The
.skip
method in Jest prevents this specific test from running, allowing you to temporarily disable the test without removing it.
Testing Error Handling in API Requests
Error handling is critical in API testing. Here’s how you can test an API that responds with an error, such as a 404 Not Found
error.
test('handles 404 error for a non-existent user', async () => {
try {
const resp= await api.get('/api/v1/project/get-all-project-by-email', {
headers:{
'Authorization': 'bearer '+ user1_authTocken
}
})
} catch (error) {
expect(error.response.status).toBe(404);
}
});
This test ensures that when a non-existent user is requested, the API returns a 404
error, and Axios throws an error that contains the correct status code.
Handling API Request Timeouts
You may want to test how your API handles request timeouts. Axios allows you to set a timeout, and you can test how it reacts when the request takes too long.
test('handles request timeout', async () => {
axios.defaults.timeout = 1000;
try {
await axios.get('/api/v1/project/get-all-project-by-email');
} catch (error) {
expect(error.code).toBe('ECONNABORTED');
}
});
This test ensures that when a request exceeds the specified timeout, Axios throws an error with the code ECONNABORTED
.
Conclusion
API testing with Jest and Axios provides a powerful combination of tools for ensuring that your web services are reliable, efficient, and handle errors correctly. By using the techniques shown in this guide, you can test API endpoints, and handle errors efficiently.