Today, almost all applications offer unique functionality for various user types (admin, manager, subscriber, guest, etc.). These groups usually are exclaim as “Roles”. Roles are the basis for permission logic; if a user has a particular role, they are able to perform that action.
CASL is a powerful library for managing access control in web applications. In a React application, CASL can be use to conditionally render components based on a user’s permissions. This allows developers to control the visibility and functionality of components based on a user’s role or other factors.
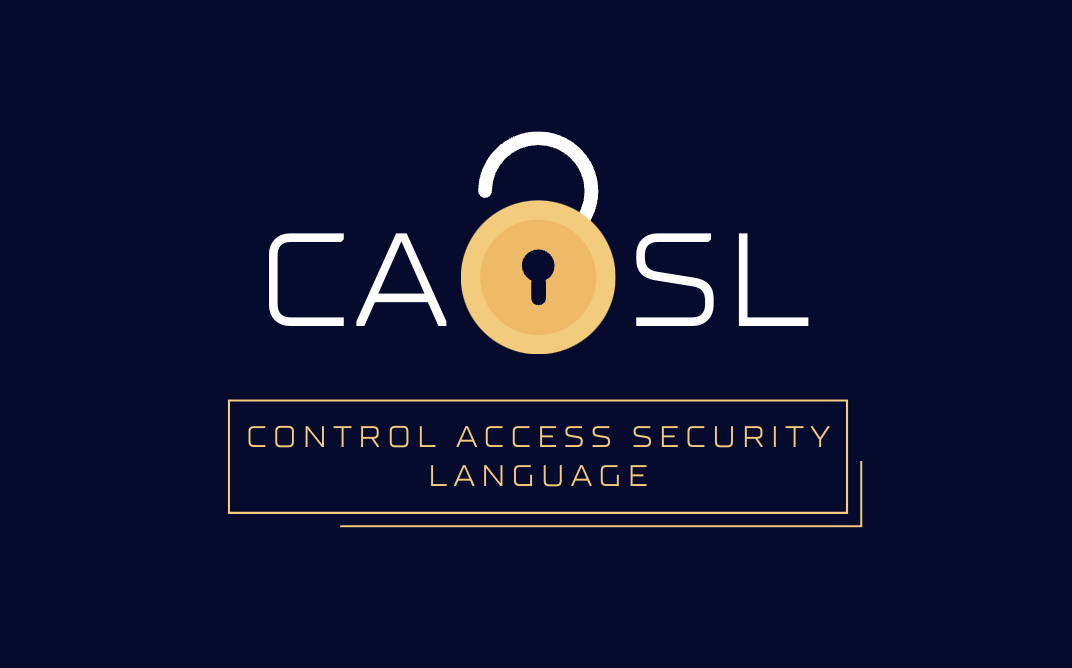
Why CASL?
Typically in a web application, users have different levels of permissions that determine what they can see and do. For example, an administrator might have access to all features of an application. While a regular user might only be able to view certain pages or perform certain actions. These permissions can be manage using CASL conditions.
How Does it Work?
For defining capabilities and examining permissions, @casl/ability uses a declarative syntax. It functions by specifying capabilities for each user role and examining those capabilities to evaluate. Whether a user is permit to carry out a specific action.
How to use CASL React conditions in a React application?
Take this as an example: in a blog application, there are two different user types: those who post blogs and those who read them. Regular users can only view blogs and comment on them; administrators can create, edit, delete, and view comments in addition to posting. Here’s how to do it:
- First, install the necessary packages using npm:
npm install @casl/react @casl/ability
@casl/ability is a npm package that provides a simple and efficient way to define abilities and check permissions in JavaScript applications.
2. Define the User’s Permissions
The first step in using @casl/ability is to define the abilities of each user role. Abilities are define using a builder function that specifies what actions each role is authorise to perform on what resources.
import { AbilityBuilder, createMongoAbility } from '@casl/ability';
import { User } from '../models'; // application specific interfaces
/**
* @param user contains details about logged in user: its id, name, email, etc
*/
function defineAbilitiesFor(user) {
const { can, cannot, build } = new AbilityBuilder(createMongoAbility);
// can read blog posts
can('read', 'Post');
// can manage own posts
can('manage', 'Post', { author: user.id });
// cannot delete a post if it is published
cannot('delete', 'Post', { isPublished: true });
return build();
});
In this example, the user has the ability to read all Posts, update the Posts that they create, and cannot delete posts that are publish.
3. Check Permissions in Components
Once you have define the user’s abilities. You can use them in your React components to check whether the user is authorise to perform a particular action.
import { Post, ForbiddenError } from '../models';
const user = getLoggedInUser(); // app specific function
const ability = defineAbilitiesFor(user)
// true if ability allows to read at least one Post
ability.can('read', 'Post');
// true if there is no ability to read this particular blog post
const post = new Post({ title: 'What is CASL?' });
ability.cannot('read', post);
// you can even throw an error if there is a missed ability
ForbiddenError.from(ability).throwUnlessCan('read', post);
In this example, by using the getLoggedInUser() function, we can obtain the user who is currently log in. This app-specific function returns the user who is currently log in.
When is define abilities function used?
The defineAbilitiesFor() function is then use to specify the user’s capabilities. This function returns an ability object that lists the user’s permitted actions after taking the logged-in user as an argument.
After defining the abilities, we can check whether the user is allow to perform certain actions. The first check uses the can() method to determine if the user can read at least one Post. The second check uses the cannot() method to determine if the user is not allow to read a specific post object.
ForbiddenError class can be used to throw an error if the user does not have the necessary abilities to read the Post object
Conclusion
@casl/ability is a powerful npm package that provides a simple and efficient way to implement role-based authorization in JavaScript applications. Developers can make sure that only approved users have access to particular features or data by defining abilities and checking permissions. @casl/ability is a great option for role-based authorization implementation in your application due to its flexible and intuitive syntax.