Integrating OpenAI with a Nest.js app involves utilizing its API for various tasks such as text completion, embeddings, image generation, and other AI services. In this guide, we will explore how to generate insights from data using Open AI’s API.
Step 1: Prepare the data
To generate insights, the input can be in various formats, including text, JSON, or other structured data. You can pass data to OpenAI in any prompt (text, JSON, etc.) depending on your use case. It’s important to format the data appropriately to get accurate insights from Open AI’s model.
Here’s an example of how we are sending the array of json data through api:
jsonData = [
{
"category": "Fresh Water",
"Date": "2024-08-03",
"value": 37.215,
"total": 102.67500000000001,
"perc_value": "36.2%"
},
{
"category": "Fresh Water",
"Date": "2024-08-04",
"value": 29.920000000000005,
"total": 79.52000000000001,
"perc_value": "37.6%"
}
]
Step 2: Obtain OpenAI API Credentials
To use Open AI’s services in your Nest.js application, you need to obtain API credentials that will allow you to authenticate and interact with Open AI’s API. Follow these steps to obtain the required API key or token:
- Sign Up for an OpenAI Account: If you haven’t done so already, visit the https://openai.com/ and sign up for an account. Once signed up, you will have access to the OpenAI dashboard.
- Access the API Section: After logging into your OpenAI account, navigate to the API section, where you’ll find detailed information about the various API services available, such as the File API, text completion API, embeddings, and more.
- Generate an API Key: In the API section, you’ll find an option to generate an API key or token. This key serves as a unique identifier, enabling your Nest.js application to securely authenticate and communicate with Open AI’s servers.
- Click on “Create new API key.”
- Once generated, make sure to copy and store the API key securely, as you will need it in your Nest.js application. OpenAI may not show it again for security reasons.
- Configure API Key in Your Application: Now that you have the API key. You’ll need to configure it in your Nest.js application. One common approach is to store the API key in environment variables to keep it secure. For example, in a
.env
file.
Step 3: Create a Request to the OpenAI API
Once JSON is ready, the next step is to send it to the backend for processing via an API call. This request will allow Open AI to analyze the data and generate insights. In the example below, we demonstrate how to dispatch the jsonData
to the backend using a function that handles the request and response flow:
import axios from 'axios';
const getOpenaiResponse = async (convertedJson) => {
try {
const response = await axios.post('/api/openai', convertedJson, {
headers: {
'Content-Type': 'application/json',
},
});
return response;
} catch (error) {
console.error('Error fetching insights:', error);
throw error;
}
};
// Call the function and handle the response
getOpenaiResponse(convertedJson)
.then((res) => {
setInsights(res?.data?.data); // Setting the response to a state variable
})
.catch((error) => {
console.error('Error fetching insights:', error);
});
In this code snippet:
axios.post('/api/openai', convertedJson)
: This sends theconvertedJson
data to your backend API, which will communicate with OpenAI for processing..then((res) => {...})
: If the API call is successful, the insights returned by OpenAI are stored in thesetInsights
state variable, which you can use to display the results in your application..catch((error) => {...})
: If an error occurs during the request, it logs the error to the console for debugging.
This setup ensures your frontend can seamlessly pass data (e.g., JSON or text) to the backend using axios
, which will then process it with OpenAI and return insights for further use in your application.
Step 4: Handling API request in the backend (Nest.js) side
To process the incoming request and interact with Open AI in your Nest.js backend, you first need to configure OpenAI’s API key. This key is essential for authenticating and authorizing your requests to OpenAI’s services.
1.Retrieve the OpenAI API Key from Configuration
Start by loading the API key from your configuration file. Using a configuration management system helps keep sensitive information like API keys secure and easily manageable.
const openAIKey = config.get("openai.key"); // Retrieve the OpenAI key from the config file
2.Initialize the OpenAI Client
After retrieving the API key, import and initialize the Open AI client in your Nest.js application. This client will handle the communication with OpenAI’s API, enabling you to send requests for text generation, embeddings, or any other AI services provided by OpenAI.
import OpenAI from "openai";
const openai = new OpenAI({
apiKey: openAIKey, // Use the retrieved OpenAI key
});
3.Interact with OpenAI for JSON Insights
Now that your JSON data is ready, it’s time to interact with the OpenAI API to generate insights. The goal is to use the OpenAI model to interpret the data and extract meaningful insights
async getOpenaiResponse(data: any) {
const dataString = JSON.stringify(data);
const prompt = `Provide a detailed insight from the below data :\n\n${dataString}\n\n-----
Put the response as a JSON object with insights in a paragraph format
\nResponse:`;
try {
let response: any; // Declare a variable to hold the response
let attempts = 0; // Counter for attempts
response = await this.openaiCreateCompletion(prompt);
const responseData = response.choices[0].message;
const responseContent = responseData.content.replace(/^```json\s+/, '').replace(/\s+```$/, '');;
const parsedContent = JSON.parse(responseContent);
const insightsArray = Object.keys(parsedContent).map(insightKey => ({
name: insightKey,
description: parsedContent[insightKey]
}));
return {
success: true,
data: insightsArray
}
} catch (error) {
console.error('Error parsing response:', error);
}
}
async openaiCreateCompletion (prompt: any) {
const chatCompletion = await openai.chat.completions.create({
model: "gpt-4-turbo-preview",
messages: [{ role: 'user', content: prompt }],
max_tokens: 1024,
});
return chatCompletion
}
- Prompt Structure: The prompt now clearly explains the task to OpenAI, asking for detailed insights and specifying the format of the response as JSON with three key insights.
- Response Parsing: The code processes the API response carefully, stripping out any code block formatting (
```json
) and then parsing it into a usable object. - Error Handling: Added more descriptive error message to help track issues if the API call fails or the response is incorrectly formatted.
- Flexibility and Clarity: The function converts the OpenAI response into an array of insights, making it easy to handle and display in your frontend.
Result
Integrating OpenAI with Nest.js can significantly enhance data analysis and improve understanding of structured information. The process involves several key steps:
- Preparing the Data: Correctly formatting the data is crucial for accurate interpretation by OpenAI’s models.
- Making API Requests: Passing the data through an API, handled on the backend, facilitates seamless communication with OpenAI.
- Handling API Requests on the Backend: Securely managing the OpenAI API key and interacting with the API in Nest.js is essential for a smooth integration.
- Generating Insights: By crafting specific prompts, OpenAI can interpret the data and return meaningful insights in a structured format, allowing for deeper understanding and easy visualization.
By following these steps, you can harness OpenAI’s AI capabilities to extract actionable insights, enhancing your application’s analytics. With Nest.js’s flexibility and OpenAI’s versatile API, you can turn raw data into valuable insights to drive decision-making and visualizations.
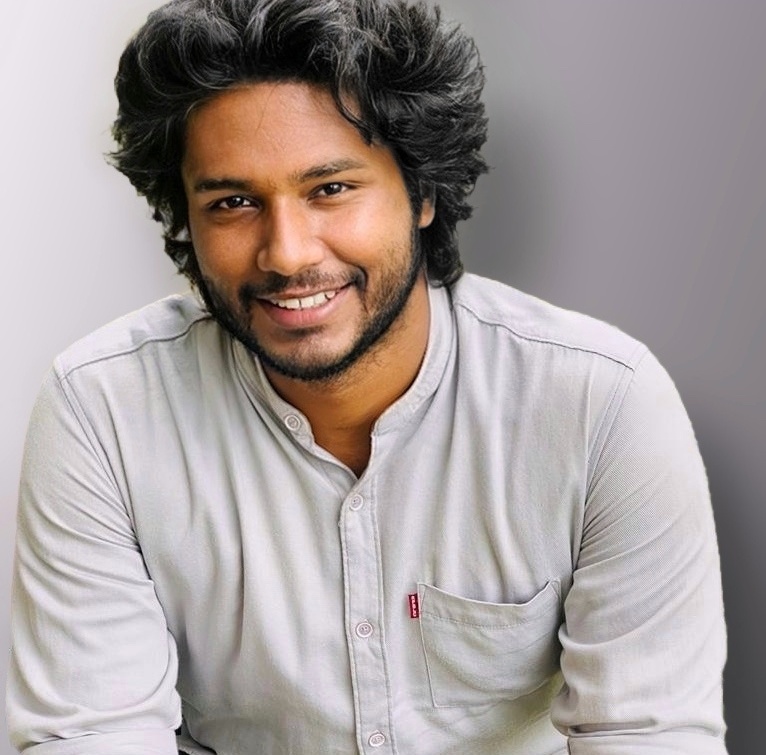
Software engineer working as a full stack developer.