Our client, a prominent leader in the sustainability space , was facing operational inefficiencies due to the use of multiple Zoho applications. While they relied on Zoho Books for financial management, Zoho Projects for project management, and Zoho Desk for customer support, these systems operated in silos. This lack of integration through Zoho API created several pain points:
- Fragmented Data: Fragmented data across finance, project, and customer support systems delayed tracking of project costs, revenue, and customer service metrics.
- Manual Effort: Employees had to manually transfer data between systems, increasing the risk of errors and leading to duplicate work.
- Delayed Decision Making: The management team struggled to access real-time insights into project profitability, customer support efficiency and financial performance due to scattered data sources.
- Scaling Challenges: As the business grew, managing these systems became even more complex without a unified view of their operations.
End Goals:
- Unified Data View: Seamlessly integrate data from Zoho Books, Zoho Projects, and Zoho Desk to allow for cross-functional reporting.
- Process Automation: Automate workflows to minimize manual intervention and errors.
- Real-time Reporting: Enable the leadership team to generate real-time reports on project progress, financial performance, and customer support metrics.
- Scalability: Create a solution that can scale as the company grows, without needing major changes or additional tools.
How We helped
As a service provider, we started by assessing the client’s current system architecture and identifying the integration points between Zoho Books, Zoho Projects, and Zoho Desk.
Get Data from Zoho Project API : A Step-by-Step Guide
Integrating Zoho Projects into your application requires proper authorization and API handling. This guide will walk you through setting up the necessary scopes, acquiring access tokens, and writing a function to fetch all projects using Zoho’s API.
Step 1: Define the Scope and Obtain the Zoho Access Key
Before making any API calls, you need to define the scope for accessing Zoho Projects. The scope determines the level of access your application will have. For this example, we will use the following scope:
Scope: ZohoProjects.projects.{Operation}
This scope allows you to perform operations (READ, CREATE, UPDATE, DELETE) on Zoho Projects. To start with, follow Zoho’s OAuth process to obtain the access key.
Step 2: Set Up Zoho API Authorization
To interact with Zoho’s API, you need to handle the authorization process. This involves obtaining and managing access tokens using the Zoho OAuth flow. Below is a code snippet that demonstrates how to generate and manage Zoho access tokens.
async getHeaders(kv = {}) {
const accessToken = await this.getAccessToken();
return Object.assign( kv, {
Authorization: `Zoho-oauthtoken ${accessToken}`,
});
}
async getAccessToken() {
if (accessTokenExires > Date.now() + 5000 && accessToken) {
return accessToken;
}
accessTokenExires = Date.now() + 3600 * 1000;
accessToken = await this.getAccessFromRefresh();
return accessToken;
}
async getAccessFromRefresh() {
return new Promise((resolve, reject) => {
const form = {
grant_type: 'refresh_token',
refresh_token: conf.ZOHO_REFRESH, //Add the refresh token in config file
client_id: conf.ZOHO_CLIENT, //Add the zoho client in config file
client_secret: conf.ZOHO_SECRET, //Add the zoho secret key in config file
};
const params = new URLSearchParams(form);
axios
.post('https://accounts.zoho.com/oauth/v2/token', params)
.then((resp) => {
resolve(resp.data.access_token);
})
.catch((ex) => {
console.error('ERROR', ex.message);
reject(ex);
});
});
}
This setup is mandatory for all Zoho APIs to perform READ, CREATE, UPDATE, and DELETE operations. The getAccessToken()
function manages the lifecycle of the access token, refreshing it when necessary.
Step 3: Fetch All Zoho Projects
With the authorization in place, you can now write a function to fetch all active projects from Zoho. The following function handles pagination and returns an array of project objects.
async getZohoProjects() {
const url = 'https://projectsapi.zoho.com/restapi/portal/[PORTALID]/projects/';
const result = [];
let index = 1;
const headers = await this.getHeaders();//Get the required headers which we added above
while (index > 0) {
//These are the params we are passing as per requirement
const params = new URLSearchParams({
status: 'active', //get the active status projects
index: index.toString(),
range: '200',
});
const { data: { projects } } = await axios.get(url, {
params,
headers,
});
for (const pr of projects) {
const ex = result.find(item => item.id_string == pr.id_string);
if (!ex) {
result.push(pr);
}
}
//Pagination
if (projects.length == 200) {
index += 200
continue
}
break;
}
return result;
}
- Authorization Headers: The
getHeaders()
function injects the required authorization token into the headers of your API request. - API Call: The
getZohoProjects()
function makes a GET request to the Zoho Projects API, retrieving active projects based on the status and pagination parameters. - Pagination: The loop fetches projects in batches of 200 until it retrieves all available projects.
- Result: The function returns an array of objects, where each object represents a single project.
This approach ensures that your application can efficiently interact with Zoho Projects, fetching all relevant data while handling the complexities of authorization and pagination behind the scenes.
Get Data from Zoho Desk API : A Step-by-Step Guide
The Zoho Desk API provides a simple and effective way to retrieve ticket information. Whether you need to fetch open tickets, closed tickets, or tickets associated with specific departments or agents, the API offers a flexible solution. In the following guide, we’ll walk you through the process of setting up the necessary authorization, making API calls, and handling the data returned by Zoho Desk to ensure you have all the information you need at your fingertips.
Step 1: Define the Scope
To begin, it’s essential to define the scope of the operations you want to perform using the Zoho Desk API. The scope determines the level of access granted to your application, ensuring that it only has the permissions necessary for the tasks at hand. Once you define the scope, obtain the Zoho access key to authenticate your API requests.
Step 2: Setup Zoho Authorization
During the authorization process, a minor adjustment is required—specifically, you need to pass an additional parameter, orgId
, to the getHeaders()
function. The orgId
represents your organization’s unique identifier within Zoho and ensures that API requests are correctly routed to your organization’s data. This step is crucial for maintaining the integrity and security of your data access.
Here’s how you can modify the getHeaders()
function to include the orgId
:
const headers = await this.getHeaders({ orgId: 'your orgId goes here' });
Step 3: Fetch All Zoho Desk Tickets
The following function demonstrates how to efficiently retrieve all tickets from Zoho Desk, including associated details like contacts, assignees, departments, teams, and products.
async getDeskTickets() {
const url = 'https://desk.zoho.com/api/v1/tickets?include=contacts,assignee,departments,team,isRead,products';
const result = [];
const headers = await this.getHeaders({ orgId: 'your orgId' });
let from = 1;
const limit = 50;
while (true) {
const params = new URLSearchParams({
from: from.toString(),
limit: limit.toString()
});
const { data } = await axios.get(url, {
params,
headers,
});
result.push(...data.data);
if (data.data.length < limit) {
break; // No more pages
}
from += limit; // Move to the next set of records
}
return result
}
- API Endpoint: The URL used in this function points to Zoho Desk’s tickets endpoint, with query parameters to include related entities such as contacts, assignees, and departments. This inclusion ensures that you get comprehensive ticket data in a single API call, reducing the need for additional requests.
- Pagination Handling: Zoho Desk API returns tickets in pages, with each page containing up to 50 tickets by default. The
from
parameter indicates the starting point for fetching records, and thelimit
defines the number of records to retrieve per request. - Looping Through Pages: The function uses a
while (true)
loop to fetch all pages of ticket data. After each request, the function checks if the number of tickets returned is less than the limit. If so, this indicates that there are no more pages left. The loop then breaks, ensuring that the function retrieves all available tickets. - Efficient Data Aggregation: The retrieved tickets are stored in the
result
array. Thepush(...data.data)
syntax appends the tickets from each page directly to this array, making it easy to manage and return the complete dataset at the end of the function. - Flexible for Large Datasets: This approach efficiently handles large datasets by automatically paginating through all available tickets without overwhelming the system with a single large request.
This function can scale and remain reliable, allowing you to effortlessly retrieve all tickets from Zoho Desk, regardless of the number of records, while keeping the code clear and organized.
ADD and DELETE Operations with Zoho Desk
In addition to fetching records, Zoho Desk API provides the flexibility to add and delete records directly through API calls. This allows for seamless management of your Zoho Desk data, whether you’re onboarding new products or removing outdated entries. Below are examples demonstrating how to add and delete products using the Zoho Desk API.
Adding Records to Zoho Desk
The following code sample illustrates how to add a new product to your Zoho Desk account. This process involves sending a POST
request to the Zoho Desk API with the necessary product details.
async addDeskProducts() {
const url = 'https://desk.zoho.com/api/v1/products'
const headers = await this.getHeaders({ orgId: 'your orgId' });
const data = {
productCode: 'Your product code',
productName: 'Your product name',
departmentIds: ["Mention your departent IDs"]
};
await axios.post(url, data, { headers });
}
- API Endpoint: The endpoint
/api/v1/products
is used to add new products to Zoho Desk. - Data Payload: The
data
object contains key details such asproductCode
,productName
, anddepartmentIds
. These fields are customizable to match your specific requirements.
Deleting Records from Zoho Desk
Deleting records is equally straightforward. The code sample below shows how to remove products by moving them to the trash. This operation is also performed via a POST
request.
async deleteZohoProducts() {
const url = 'https://desk.zoho.com/api/v1/products/moveToTrash'
const headers = await this.getHeaders({ orgId: 'your OrgId' });
const data = {
productIds : [ 'Your product IDs' ]
};
const response = await axios.post(url, data, { headers });
console.log(`Product deleted successfully`, response.data);
}
- API Endpoint: The endpoint
/api/v1/products/moveToTrash
is used to delete products from Zoho Desk by moving them to the trash. - Data Payload: The
data
object contains theproductIds
that specify which products should be deleted.
Get Data from Zoho Books API : A Step-by-Step Guide
When it comes to managing your financial data, integrating Zoho Books with your existing systems can be a game-changer. Zoho Books is a comprehensive cloud accounting solution that allows businesses to manage their finances, automate business workflows, and stay tax compliant. To harness the full potential of Zoho Books, you can leverage its powerful API to fetch, update, and manage your financial data programmatically.
In this guide, we’ll walk you through the steps required to set up and use the Zoho Books API, from obtaining the necessary authorization to making API calls that retrieve detailed financial records, such as invoices, payments, and transactions. This process involves several key steps, each designed to ensure secure and efficient access to your financial data.
Here’s a breakdown of the essential steps:
Define the Scope and Obtain Authorization:
Just like with other Zoho APIs, you need to define the appropriate scopes to ensure that your application has the necessary permissions to access specific modules in Zoho Books. Once you define the scope, obtain an OAuth access token to authenticate your API requests.
Set Up API Headers:
The Zoho Books API requires specific headers for authentication. These headers include your OAuth token and any additional parameters needed. Setting up these headers correctly is crucial for successful API calls.
Fetching Data through books API:
Once your authorisation and headers are in place, you can start fetching data from Zoho Books. This could include a variety of financial records such as invoices, customers, vendors, transactions, and more.
Below is a sample code snippet that demonstrates how to fetch project data from Zoho Books using the API:
async getProjectsFromZohoBooks() {
const url = 'https://www.zohoapis.com/books/v3/projects';
const headers = await this.getHeaders();
const result = [];
let page = 1;
while (page > 0) {
const params = new URLSearchParams({
page: page.toString(),
organization_id: 'xxxxxxx', //Your Organisation ID
filter_by: 'Status.Active',
range: '200',
});
const { data: { projects, page_context } } = await axios.get(url, {
params,
headers,
});
for (const pr of projects) {
result.push(pr);
}
if (!page_context.has_more_page) {
break;
}
page++;
}
return result;
}
Key Consideration:
- Pagination Handling:
The API may return data in a paginated format, especially when dealing with large datasets. This code loops through each page to retrieve all the data.
- Data Filtering:
Thefilter_by
parameter is used to filter the data. In this example, the code fetches only projects with an active status. You can adjust this filter based on your specific requirements.
- Organization ID:
Theorganization_id
parameter is mandatory and needs to be replaced with your actual Organization ID. This ID ensures that the data fetched is specific to your organization.
What we achieved
- Efficiency Gains: Employees reduced the manual effort of transferring data between systems by 80%, allowing them to focus on higher-value tasks.
- Real-time Insights: The management team could now track project profitability and customer support performance in real-time, enabling faster decision-making.
- Improved Collaboration: With unified data, finance, project management, and customer support teams were able to collaborate more effectively, enhancing overall operational efficiency.
- Scalability: The integration architecture was designed to scale, meaning the company could continue using the same system as they expanded, without needing further significant investments in software.
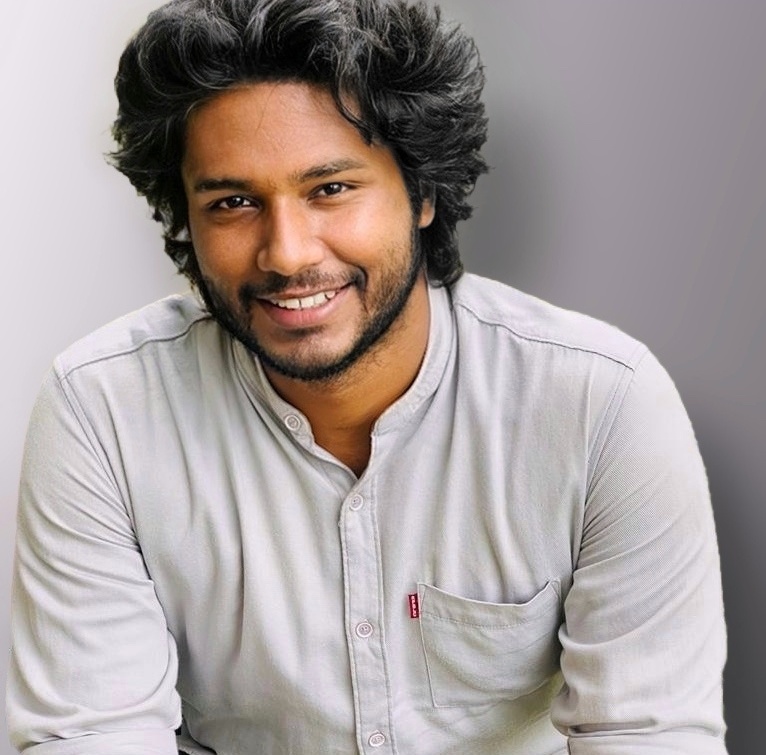
Software engineer working as a full stack developer.